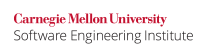
Methods can return values to communicate failure or success or to update local objects or fields. Security risks can arise when method return values are ignored or when the invoking method fails to take suitable action. Consequently, programs must not ignore method return values.
When getter methods are named after an action, a programmer could fail to realize that a return value is expected. For example, the only purpose of the ProcessBuilder.redirectErrorStream()
method is to report via return value whether the process builder successfully merged standard error and standard output. The method that actually performs redirection of the error stream is the overloaded single-argument method ProcessBuilder.redirectErrorStream(boolean)
.
Noncompliant Code Example (File Deletion)
This noncompliant code example attempts to delete a file but fails to check whether the operation has succeeded:
public void deleteFile(){ File someFile = new File("someFileName.txt"); // Do something with someFile someFile.delete(); }
Compliant Solution
This compliant solution checks the Boolean value returned by the delete()
method and handles any resulting errors:
public void deleteFile(){ File someFile = new File("someFileName.txt"); // Do something with someFile if (!someFile.delete()) { // Handle failure to delete the file } }
Noncompliant Code Example (String Replacement)
This noncompliant code example ignores the return value of the String.replace()
method, failing to update the original string. The String.replace()
method cannot modify the state of the String
(because String
objects are immutable); rather, it returns a reference to a new String
object containing the modified string.
public class Replace { public static void main(String[] args) { String original = "insecure"; original.replace('i', '9'); System.out.println(original); } }
It is especially important to process the return values of immutable object methods. Although many methods of mutable objects operate by changing some internal state of the object, methods of immutable objects cannot change the object and often return a mutated new object, leaving the original object unchanged.
Compliant Solution
This compliant solution correctly updates the String
reference original
with the return value from the String.replace()
method:
public class Replace { public static void main(String[] args) { String original = "insecure"; original = original.replace('i', '9'); System.out.println(original); } }
Risk Assessment
Ignoring method return values can lead to unexpected program behavior.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
EXP00-J | Medium | Probable | Medium | P8 | L2 |
Automated Detection
Tool | Version | Checker | Description |
---|---|---|---|
CodeSonar | 8.1p0 | JAVA.NULL.RET.UNCHECKED | Call Might Return Null (Java) |
Coverity | 7.5 | CHECKED_RETURN | Implemented |
Parasoft Jtest | 2023.1 | CERT.EXP00.NASSIG CERT.EXP00.AECB | Ensure method and constructor return values are used Avoid "try", "catch" and "finally" blocks with empty bodies |
PVS-Studio | 7.30 | V6010, V6101 | |
SonarQube | 9.9 | Return values from functions without side effects should not be ignored Return values should not be ignored when they contain the operation status code | |
SpotBugs | 4.6.0 | RV_RETURN_VALUE_IGNORED | Implemented |
Related Guidelines
VOID EXP12-CPP. Do not ignore values returned by functions or methods | |
Passing Parameters and Return Values [CSJ] | |
CWE-252, Unchecked Return Value |
Bibliography
[API 2006] | |
Misusing | |
[Seacord 2015] |
6 Comments
David Svoboda
As in C, many methods return values that can be safely discarded or ignored...how does this rec deal with them?
Dhruv Mohindra
I think the purpose of this recommendation is to make sure the programmer checks the API documentation and makes his/her own choice about whether the return value is to be discarded or not. That's why it says 'do not ignore the value' and not 'do not discard the value'. What is your opinion, otherwise?
David Svoboda
The first NCCE/CS is vulnerable to a TOCTOU race condition...what if an attacker manipulates the filesystem so that a sensitive file has the name of the file to be deleted? That is, an attacker can trick the JVM into deleting a different file than it is performing operations on.
(FWIW the remove() function in C99 has the same functionality and the same vulnerability.)
Robert Seacord
is there a way for a programmer to indicate they intentionally chose to ignore a return value, for example, casting to (void) in C?
David Svoboda
The C trick of adding a
(void)
typecast doesn't compile in Java. AFAIK the community does not provide a way to indicate a function's value is ignored. I suppose one could create an annotation to handle this, but I don't know of one offhand.Robert Seacord (Manager)
Somehow I think these examples could be more compelling. Perhaps the string replacement replaces command separation characters in a command string, or the file being deleted contains sensitive information?