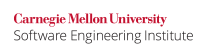
The contracts of the read methods for the InputStream
family are complicated. According to the Java API [API 2006] for the class InputStream
, the read(byte[], int, int)
method provides the following behavior:
The default implementation of this method blocks until the requested amount of input data len has been read, end of file is detected, or an exception is thrown. Subclasses are encouraged to provide a more efficient implementation of this method.
However, the read(byte[])
method states that it:
Reads some number of bytes from the input stream and stores them into the buffer array
b
. The number of bytes actually read is returned as an integer. The number of bytes read is, at most, equal to the length ofb
.
Note that the read()
methods return as soon as they find available input data. Ignoring the result returned by the read()
methods is a violation of rule EXP00-J. Do not ignore values returned by methods. Security issues can arise even when return values are considered, because the default behavior of the read()
methods lacks any guarantee that the entire buffer array will be filled. The programmer must check the number of bytes actually read and call the read()
method again as required.
Noncompliant Code Example (read()
)
This noncompliant code example attempts to read 1024
bytes encoded in UTF-8
from an InputStream
and to return them as a String
. It explicitly specifies the the encoding to build the string, in compliance with IDS17-J. Use compatible encodings on both sides of file or network IO.
public static String readBytes(InputStream in) throws IOException { byte[] data = new byte[1024]; if (in.read(data) == -1) { throw new EOFException(); } return new String( data, "UTF-8"); }
The programmer's misunderstanding of the general contract of the read()
methods can result in failure to read the intended data in full...it is possible for the data to be less than 1024 bytes long, with additional data available from the InputStream
.
Compliant Solution (Multiple calls to read()
)
This compliant solution reads all the desired bytes into its buffer, accounting for the total number of bytes read and adjusting the remaining bytes' offset, thus ensuring that the required data are read in full. It also avoids splitting multibyte encoded characters across buffers by deferring construction of the result string until the data have been read in full, see IDS13-J. Do not assume every character in a string is the same size for more information.
public static String readBytes(InputStream in) throws IOException { int offset = 0; int bytesRead = 0; byte[] data = new byte[1024]; while (true) { bytesRead += in.read(data, offset, data.length - offset); if (bytesRead == -1 || offset >= data.length) break; offset += bytesRead; } String str = new String(data, "UTF-8"); return str; }
Compliant Solution (readFully()
)
The no-argument and one-argument readFully()
methods of the DataInputStream
class guarantee that they either will read all of the requested data or will throw an exception. These methods throw EOFException
if they detect the end of input before the required number of bytes have been read; they throw IOException
if some other input/output error occurs.
public static String readBytes(FileInputStream fis) throws IOException { byte[] data = new byte[1024]; DataInputStream dis = new DataInputStream(fis); dis.readFully(data); String str = new String(data, "UTF-8"); return str; }
Risk Assessment
Failure to comply with this rule can result in the wrong number of bytes being read or character sequences being interpreted incorrectly.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
FIO02-J |
low |
unlikely |
medium |
P2 |
L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Bibliography
[[API 2006]] Class InputStream
, DataInputStream
[[Chess 2007]] 8.1 Handling Errors with Return Codes
[[Harold 1999]] Chapter 7: Data Streams, Reading Byte Arrays
[[MITRE 2009]] CWE ID 135 "Incorrect Calculation of Multi-Byte String Length"
[[Phillips 2005]]
FIO01-J. Do not expose buffers created using the wrap() or duplicate() methods to untrusted code 12. Input Output (FIO) FIO03-J. Create files with appropriate access permissions